A while ago I, I found this awesome tiny Arduino with embedded display on Kickstarter. After some waiting, the cute little device landed on my doormat. And although I instantly fell in love, I didn’t really had the time to use it in a nice project. Until today …
Yesterday, I figured this device was a perfect little friend to function as my Mac’s second screen. I managed to create a nice sketch that takes any string from the serial port, and display it as a tickertape on the 64x48 pixel OLED screen.
This way, I can use ANY bash script that outputs a string, to display it on my Micro Second Screen. It allows me to show my Mac’s uptime and load, or even recent #arduino tweets.
How this is done? Let’s check the Arduino Sketch first …
The Sketch
First off, we need to include the MicroView library and define some contants and variables:
// MicroViewSecondScreen.ino
#include <MicroView.h>
// Define the settings
#define Y_POS 18 // Vertical text position.
#define START_POS 63 // Horisontal start position
#define SPEED 10 // Speed of the animation. Lower is faster.
#define FONT_TYPE 1 // Set the type of the font
// Define some helper variables.
int basePosition=START_POS;
int characterWidth=0;
String contentString = "Waiting for data ..."; // Contains the string currently displayed.
String displayString = contentString; // Contains the next string to display.
boolean returnFound = true;
boolean newString = true;
char character;
Now it’s time to setup the Arduino in the setup()
function:
void setup() {
// Set up the screen
uView.begin();
uView.clear(ALL);
uView.display();
uView.setFontType(FONT_TYPE);
// Get the character size for later use.
characterWidth=uView.getFontWidth();
// Open the the serial port.
Serial.begin(9600);
while (!Serial);
Serial.print("Serial port ready.");
// Wait half a second before we start the animation.
delay(500);
}
With the setup function in place, let’s construct the loop()
function:
void loop() {
// Check the serial port for new data
readSerial();
// Update the screen to the new animation state.
updateScreen();
// Remain in this state for x microseconds.
delay(SPEED);
// Check if we need to update the message.
updateMessage();
}
In the above function we use three custom functions, from which the first is readSerial()
. This function checks the serial port for new data.
void readSerial() {
//As long as there is new data available, run the following code.
while(Serial.available()) {
// If, in a previous run, we found a return. Reset the full string.
if (returnFound) {
contentString = "";
returnFound = false;
}
// Read one character and add it to the contentString.
character = Serial.read();
contentString.concat(character);
// If we receive a return, toggle the returnFound and newString to true.
if (character == '\n') {
returnFound = true;
newString = true;
}
}
}
Of course, we need the function that does all the screen drawing: updateScreen()
. This function will use a helper function called drawChar(int x, int y, uint8_t value)
.
void updateScreen() {
// Clear the string.
uView.clear(PAGE);
// Get the length of the string.
int stringLength = displayString.length();
// Loop thru all the characters in the displayString.
for (int characterIndex=0; characterIndex<stringLength; characterIndex++){
// Draw the character on the appropriate location.
drawChar(basePosition+characterWidth*characterIndex,Y_POS,displayString[characterIndex]);
}
// Decrease the base position for the next update, making the tekst scroll.
basePosition--;
// Update the screen after everything above is done.
uView.display();
}
void drawChar(int x, int y, uint8_t value) {
// Check if the character we want to draw is within the visible bounds.
if ((x + characterWidth > 0) && (x < 64)) {
// If so, draw it to the screen.
uView.drawChar(x,y,value);
}
}
And this leaves us with only one function: updateMessage()
to check if there is a new string to display:
void updateMessage() {
// Get the length of the string.
int stringLength = displayString.length();
// Check if we scrolled all the way thru the string.
if (basePosition < -stringLength * characterWidth) {
// If so, reset the bese position to the start position.
basePosition=START_POS;
// Check if there is a new message available.
if (newString) {
// If so, set the new displayString.
displayString = contentString;
// Reset the newString variable.
newString = false;
}
}
}
With above sketch in place, the Arduino takes any string on from the serial port, and shows it on the screen like this:
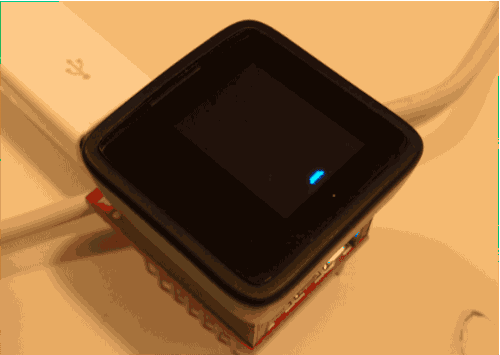
Sending strings to the MicroView.
With the Arduino connected to your Mac, It’s pretty simple to send any string to your Tiny Second Screen:
echo 'Hello World' >> /dev/tty.usbserial-DA00U91G
In this case, the /dev/tty.usbserial-DA00U91G
part is the path name to the serial port. Check your Arduino IDE to figure out the right serial port.
With this in mind, we can write small Bash script to display the time …
while true; do echo `uptime` >> /dev/tty.usbserial-DA00U91G; sleep 5; done;
… your Mac’s uptime …
while true; do echo `uptime` >> /dev/tty.usbserial-DA00U91G; sleep 5; done;
… or even the most recent #arduino tweets!
while true; do curl http://michmich.com/lasttweet/?q=%23arduino\&num=5 >> /dev/tty.usbserial-DA00U91G; sleep 20; done;
If you’re creative you can even show Spotify’s current track information by using shpotify. But I’ll leave that one for you to figure out.
Let me know if you find a fun way to use your MicroViewSecondSreen! Leave a comment down below.
As always, the full Sketch is available on GitHub.